What is Overloading and Overriding?
We read overloading and overriding separately so that we are easy to understand. So we start with overloading first
What is overloading?
But to address the individuals we use first name along with last name to uniquely identify that individual.
Overloading is quite similar to what we saw above.
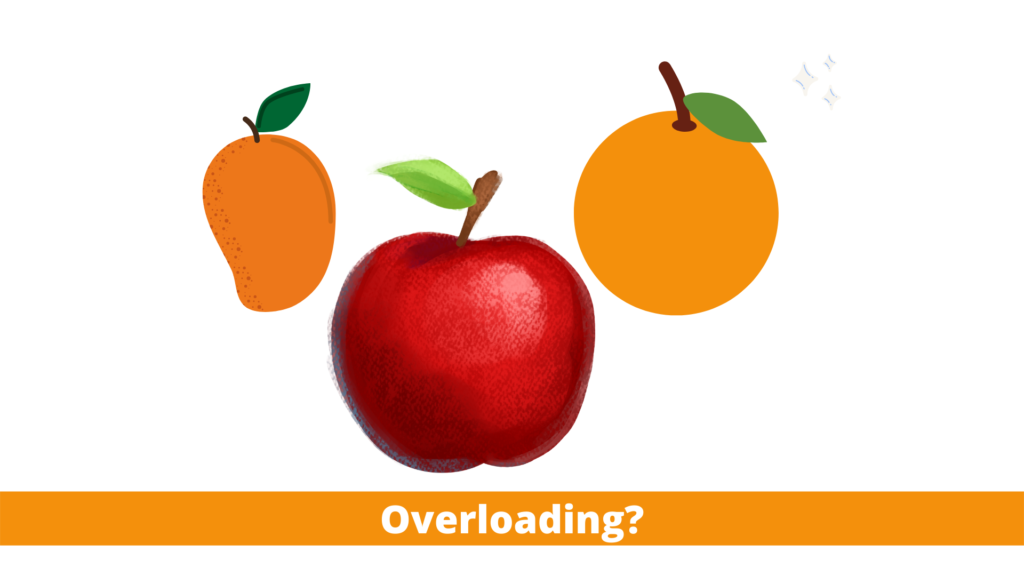
Overloading is when two or more methods in a class have same name.
But have different parameters.
The parameters can differ with respect to the number of parameters or type.
But, I can write multiple methods doing the same task with different parameters.
Seriously? Why?
Overloading prevents us from writing multiple methods which perform the same task.
We can overload the methods and manipulate the type of parameters that they accept
Lets see this in action.
class Main {
public static void dontOverloadMe(){
System.out.println(“Please, don’t.”);
}
public static void dontOverloadMe(String laugh){
System.out.println(“Oops! Couldn’t hold it for long. “+ laugh);
}
}
In class Main, we have two methods with same name, one of which accepts no parameters and another one which accepts one parameter.
Thus, we can say that the second method overloads the first one.
But how to identify which one is called when?
The compiler does that for you.
When you call a method it checks for the parameters passed if any and accordingly executes the respective method.
Let’s try calling the overloaded method.
class Main {
public static void dontOverloadMe(){
System.out.println(“Please, don’t.”);
}
public static void dontOverloadMe(String laugh){
System.out.println(“Oops! Couldn’t hold it for long. “+ laugh);
}
public static void main(String[] args) {
dontOverloadMe();
//calling the function without any parameters
}
}
The output of the above code is:
Please, don’t.
Awesome! But what if I passed a parameter with it?
class Main {
public static void dontOverloadMe(){
System.out.println(“Please, don’t.”);
}
public static void dontOverloadMe(String laugh){
System.out.println(“Oops! Couldn’t hold it for long. “+ laugh);
}
public static void main(String[] args) {
dontOverloadMe(“HaHaHa”); //calling the function with a parameter
}
}
The output of the above snippet will be
Oops! Couldn’t hold it for long. HaHaHa
Hell, Yeah! That’s how it is done.
What is Overriding?
Some members of a family have a nickname as the real name is very long or hard to call.
Thus, in this case nickname is being used to identify the same individual.
Overriding aligns perfectly with the above example.
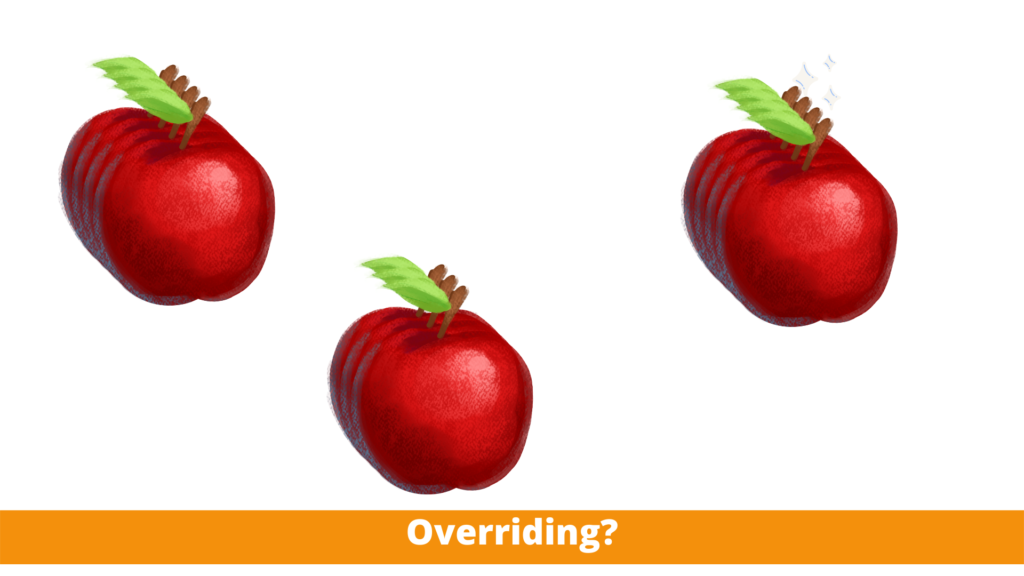
Overriding is when two or more methods in separate classes having child-parent relationship have the same name.
And also same parameters.
But, the two methods are not written in the same class.
The method which overrides the previous one is written in a child class.
This enables us to provide specifications while implementing the same function in a child class.
We have already learned about child classes and inheritance.
It is important to note that overriding takes place when a method declared in the parent class is rewritten in a child class with same parameters.
Let’s see how overriding is implemented.
class ParentClass{
public void oldMethod(){
System.out.println(“Parents rock.”);
}
}class ChildClass extends ParentClass{
public void oldMethod(){
System.out.println(“Children rock. “);
}
}
Here, ChildClass is a class inherited from ParentClass which contains the same function oldFunction() declared in ParentClass.
According to you what will happen if we call the method oldMethod?
class ParentClass{
public void oldMethod(){
System.out.println(“Parents rock.”);
}
}class ChildClass extends ParentClass{
public void oldMethod(){
System.out.println(“Children rock.”);
}
}
public class Main{
public static void main(String [] args){
ParentClass obj1 = new ChildClass();
obj1.oldMethod();
//calling method oldMethod
}
}
Can you try to guess the output of above snippet?
Children rock.
To Summarize
- Overloading is when two or more methods in a class have the same name but different parameters.
- Overloading prevents us from writing multiple methods which performs the same task.
- Overriding is when two or more methods in a class have the same name and same parameters.
- The method which overrides the previous one is written in a child class.