What is encapsulation?
Imagine you have a water bottle which is filled with water upto the brim.
There are two marbles present inside the water bottle.
Can you pull these marbles out without spilling the water?
What if you are given a pair of tongs?
You can, right?
Encapsulation is a concept in Object Oriented Programming which describes the idea of bundling variables and methods together.
It brings the idea of data hiding to the table.
The variables in the class can only be accessed through the methods declared inside the class.
The variables are declared as private.
And the methods to access them as public so that they can be accessed from outside the class.
Then only these methods can be used to set or get the values of the variables.
Encapsulation provides a protected space for data members and restricts access outside the class.
Let’s see how we can achieve encapsulation in Java.
public class Student{
private String name; //private data members
private int roll_no;
public void setName(String newName){
//public methods
name = newName;
}
public void setRollNo(int roll){
roll_no = roll;
}
}
In the above written code, we can see that name and roll_no are declared as private therofore they can’t be accessed outside the class.
But the methods setName() and setRollno() are declared as public.
Thus, these methods can be accessed from outside the class.
We can call these methods and pass the values of newName and roll which are then assigned to the name and roll_no variables.
Thus we cannot change the values of these variables without accessing those methods.
//File: Student.java
public class Student{
private String name; //private data members
private int roll_no;
public void setName(String newName){
//public methods
name = newName;
}
public void setRollNo(int roll){
roll_no = roll;
}
}
//File: Main.java
public class Main {
public static void main(String[] args) {
Student obj1 = new Student();
obj1.setName(“Rocket”);
//Calling method setName
}
}
Here,we are calling the method setName and setting the value of the variable name.
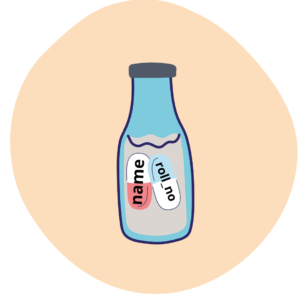
The variables name and roll_no are declared as private and hence cannot be accessed.
Thus, we have public methods setName and setRollNo to assign values to these variables.
Here, we have bundled variables and methods together, which is nothing but encapsulation.
Let’s try to define another method which displays the value of name.
//File: Student.java
public class Student{
private String name;
public void setName(String newName){
name = newName;
}
public void dispName(){
System.out.println(name);
}
}
//File: Main.java
class Main {
public static void main(String[] args) {
Student obj1 = new Student();
obj1.setName(“Rocket”);
obj1.dispName();
}
}
Can you try to guess the output of the above snippet?
Here we have a method dispName() which displays the value of variable name.
After we call the setName() method with Rocket as the parameter we are calling the dispName() method.
Thus, the output of the snippet will be
Rocket
To summarize
- Encapsulation is a concept in OOP which describes the idea of bundling variables and methods together.
- The variables are declared as private.
- And the methods to access them as public so that they can be accessed from outside the class.
- Encapsulation provides a protected space for data members and restricts access outside the class.